일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | ||||||
2 | 3 | 4 | 5 | 6 | 7 | 8 |
9 | 10 | 11 | 12 | 13 | 14 | 15 |
16 | 17 | 18 | 19 | 20 | 21 | 22 |
23 | 24 | 25 | 26 | 27 | 28 |
- glfw
- git
- 프로젝트 여러개
- Tutorial
- 솔루션에프로젝트추가
- 시작
- .sln
- fetching
- visualstudip
- vertex sahder
- 파일생성 명령어
- vertexarrayobject
- 튜링기계
- 자동판결
- shader
- Qtspim
- OpenGL
- interface block
- fragment
- superbible
- qtspim stack
- 멀티프로세스
- multi process
- 정의
- 하나의 솔루션
- VAO
- glDrawArrays
- vertex
- turingmachine
- 수리명제
- Today
- Total
공사중
strcpy, sizeof(char*)에 대한 고찰 본문
strcpy를 사용하던 중 다음과 같은 두 개의 의문이 들었습니다.첫 번째, 아래 코드에서는 LEN의 크기는 아무런 상관없이 strcpy_s의 두 번째 인자의 크기가 세 번째 인자의 크기보다 크면 문자열의 복사가 가능하다는 것.
#include <iostream> #include <cstring> using namespace std; #define LEN 10 //LEN의 크기와 상관없이 strcpy_s가 에러없이 작동한다. int main(void) { char name[5] = "1234"; char *strPtr = new char[LEN]; strcpy_s(strPtr,strlen(name)+1,name); cout << strPtr << endl; cout << strlen(strPtr) << endl; return 0; }
위 예제에서는 LEN의 크기와 상관없이 strcpy_s가 에러없이 작동했습니다.하지만 아래 예제에서는 LEN의 크기가 name의 길이보다 +1이상 커야합니다.
#include <iostream> #include <cstring> using namespace std; #define LEN 5 int main(void) { char name[5] = "1234"; char dynamic[LEN] = { 'a','b' }; strcpy_s(dynamic, strlen(name) + 1, name); cout << dynamic << endl; cout << strlen(dynamic) << endl; return 0; }
제가 찾은 원인은 이렇습니다.strcpy_s reference에는 다음과 같이 나와있습니다. Copies the null-terminated byte string pointed to by src, including the null terminator, to the character array whose first element is pointed to by dest.즉, 동적배열의 크기와 상관없이 dest가 그저 배열이기만 하면 되는 것입니다.그러나!The behavior is undefined if the dest array is not large enough. dest의 크기가 충분하지 않다면 strcpy_s가 정의되지 않아서 에러가 납니다.new를 사용했을 때 dest의 크기가 부족하게 할당하더라도 버퍼에러가 뜨지 않는 이유는 아래와 같습니다.https://stackoverflow.com/questions/22008755/how-to-get-size-of-dynamic-array-in-c
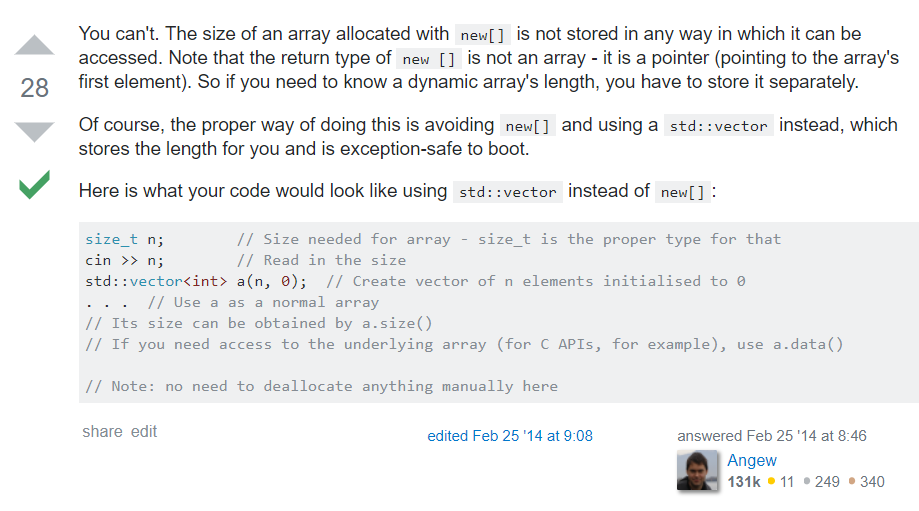
바로 동정 배열의 크기를 그 포인터만으로는 알 수가 없기 때문입니다.위에서 Angew가 언급한 것과 같이 이러한 이유로 동적할당의 크기를 기억하고 있거나, vector를 사용해야합니다.
두 번째, 이것은 고찰보다는 제가 잘못알고 있었던 부분을 바로 잡는 것에 가깝습니다.
#include <iostream> #include <cstring> using namespace std; int main(void) { cout << "sizeof char is..."<< sizeof(char) << endl; //1 cout << "sizeof char* is..." << sizeof(char*) << endl; //4 cout << "sizeof int is..." << sizeof(int) << endl; //4 cout << "sizeof int* is..." << sizeof(int) << endl; //8 cout << "sizeof float is..." << sizeof(float) << endl; //포인터는 항상 크기가 4 cout << "sizeof float* is..." << sizeof(float*) << endl; //포인터는 항상 크기가 4 cout << "sizeof double is..." << sizeof(double) << endl; //포인터는 항상 크기가 4 cout << "sizeof double* is..." << sizeof(double*) << endl; //포인터는 항상 크기가 4 return 0; }
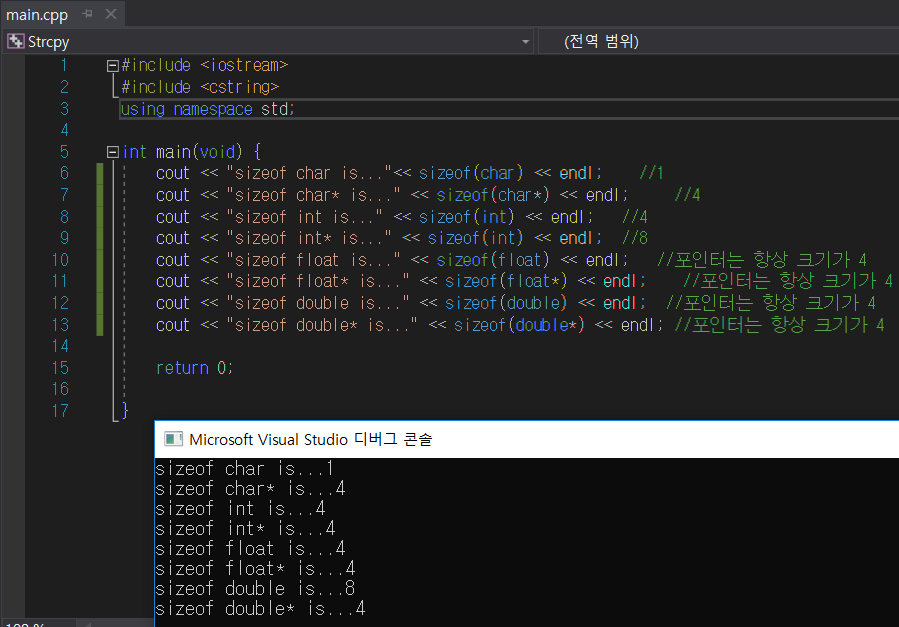
#include "stdio.h" int main(void) { printf("sizeof char is...%d\n", sizeof(char)); printf("sizeof char* is...%d\n", sizeof(char)); printf("sizeof int is...%d\n", sizeof(int)); printf("sizeof int* is...%d\n", sizeof(int*)); printf("sizeof float is...%d\n", sizeof(float)); printf("sizeof float* is...%d\n", sizeof(float*)); printf("sizeof double is...%d\n", sizeof(double)); printf("sizeof double* is...%d\n", sizeof(double*)); return 0; }
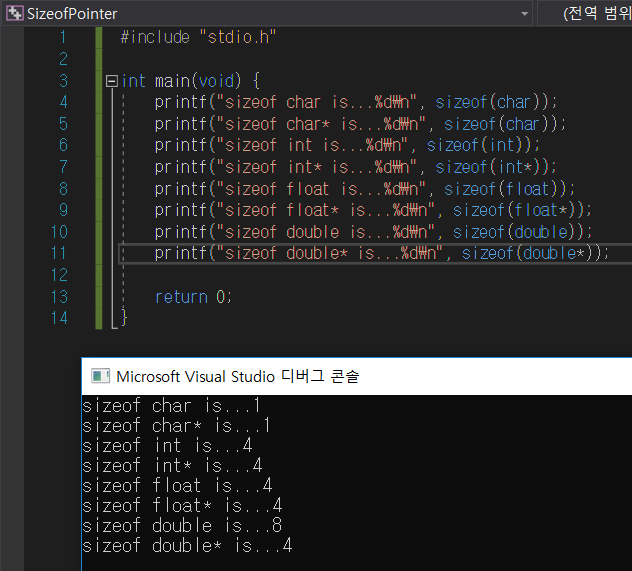
'개발 | C++' 카테고리의 다른 글
[Visual studio] 솔루션과 프로젝트 (1) | 2019.03.01 |
---|---|
(int++)++가 안되는 이유 (0) | 2019.02.18 |
C++기본 syntax는... (0) | 2019.02.18 |